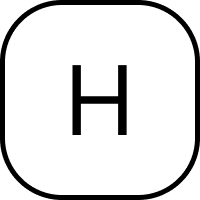
Hemant Bhatt
SEO Meets UX: Mastering Next.js Streaming, Suspense and Loading
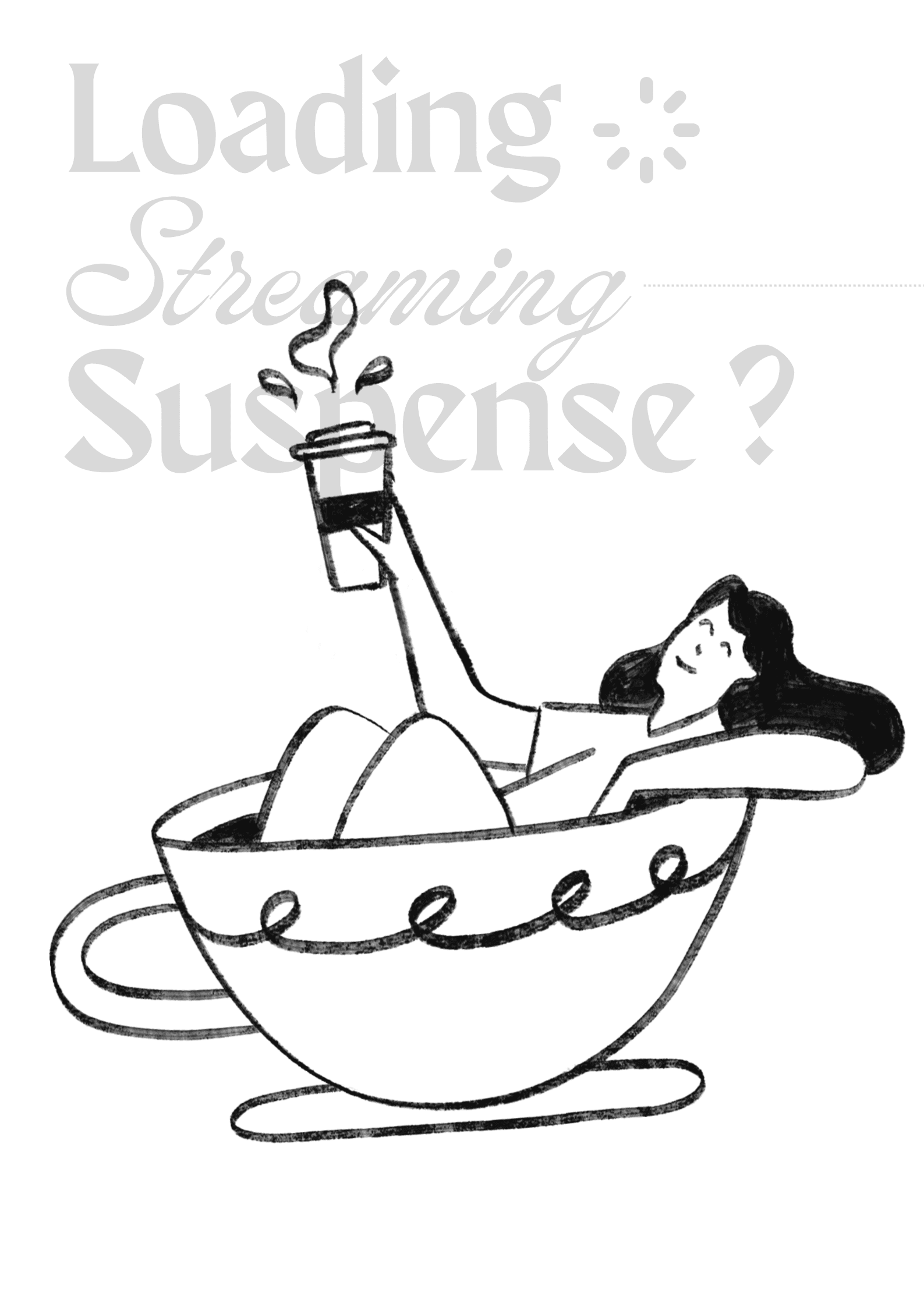
Introduction
Rendering pages can be a complicated affair. While your servers are busy chatting with databases and performing digital gymnastics, your users are tapping their fingers impatiently on their desks. Multiple research confirms what we already suspected. Spinners and skeleton screens work wonders during wait times. Serving partial data or loading states preserves something more precious than gold... “User Engagement”.
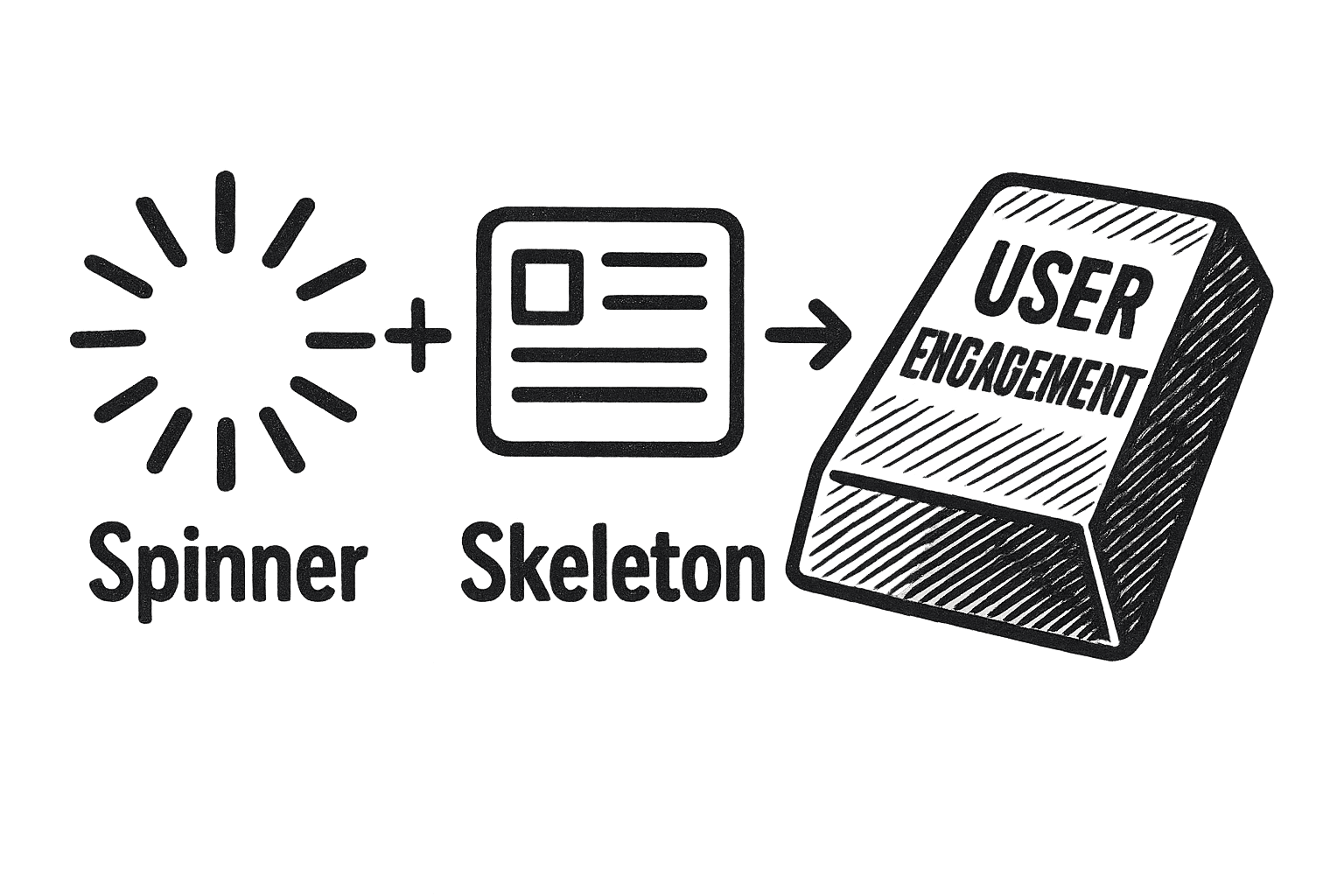
The two ways to handle loading in Nextjs
- Directory wise: When you place a loading.(tsx/jsx) file in the same directory as your page component, Next.js automatically uses it as a fallback while your page loads. Think of it as your page's personal assistant, entertaining the audience while the main act gets ready backstage. For example, if your page.tsx is busy fetching data or performing server operations, the component exported from loading.(tsx/jsx) will be displayed until everything's ready. No more awkward blank screens or users wondering if your app crashed! This directory-based approach means you can customize loading states for each route in your application with minimal effort. It's like having a different waiting room for each doctor in a clinic – much nicer than one generic waiting area for everyone.
- Component wise: While loading.tsx is useful, it has one significant drawback: it blocks your entire page until everything is ready. Not ideal when just one component is the slowpoke. Imagine hosting a dinner party where everyone has to wait outside until the slowest dish finishes cooking. Pretty awkward, right? Component-level streaming solves this problem by letting you isolate and handle slow-loading parts individually. Here's how: Identify your performance bottlenecks (those components with lengthy data fetching). Wrap them in React's Suspense component with an appropriate fallback. Let the rest of your page load normally.
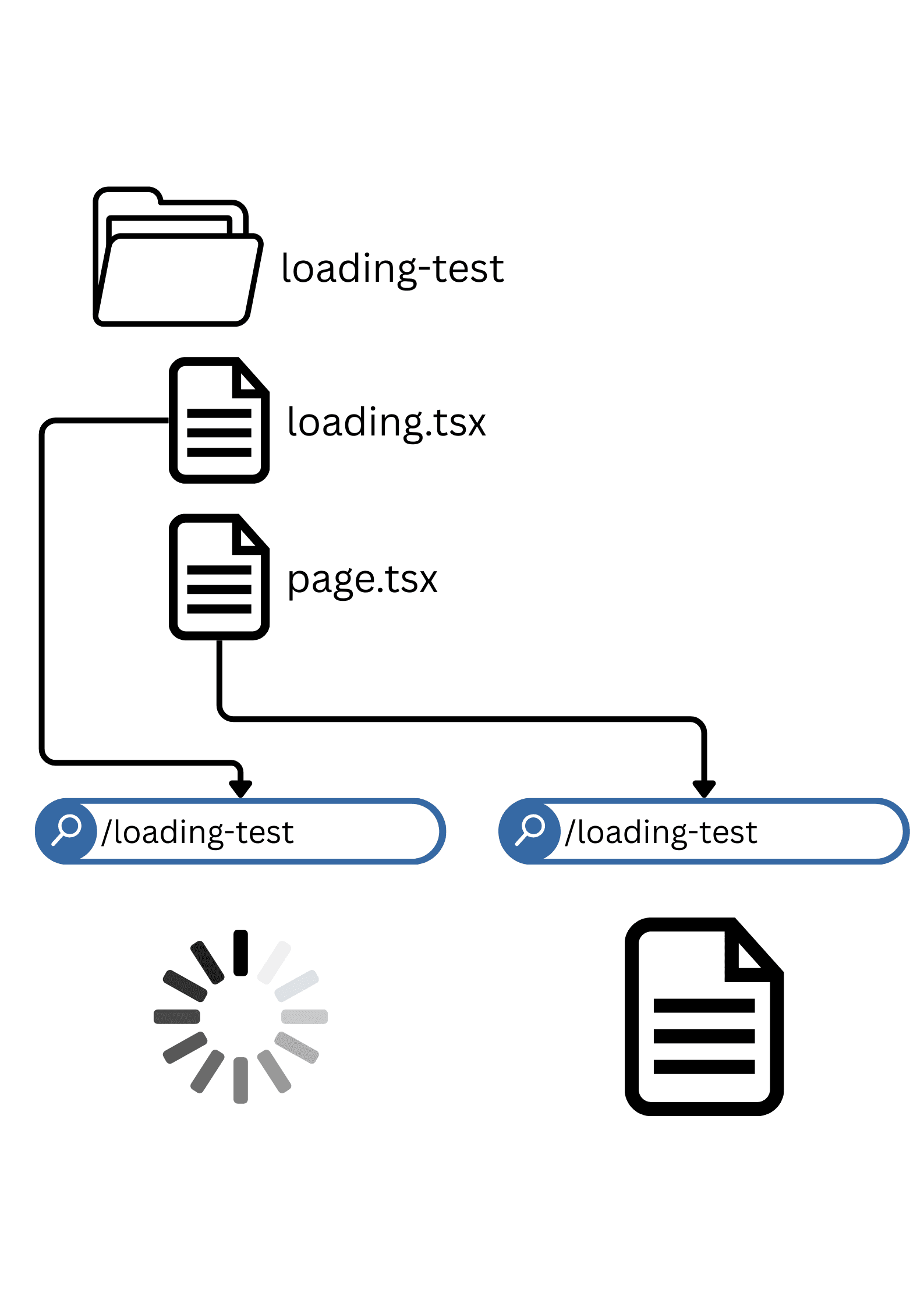
1 export default function Page() { 2 return ( 3 <div> 4 <ImportantComponent /> 5 <Suspense fallback={<Spinner />}> 6 <SlowComponent /> 7 </Suspense> 8 <FastComponent /> 9 <Suspense fallback={<LoadingSkeleton />}> 10 <VerySlowComponent /> 11 </Suspense> 12 </div> 13 ); 14 }
This approach delivers the speedy parts of your page immediately while showing fallback content (like spinners or skeletons) for the slower components. When each slow component finishes, it streams in seamlessly. The result? Better Time to First Byte (TTFB) and First Contentful Paint (FCP) metrics—and happier users who don't feel like they're watching digital paint dry. Also, you get to choose which component’s loader looks how.
SEO and Suspense: Does Google See Your Streamed Content?
A common worry with streaming: "If I show loading UI first, will search engines miss my actual content?" Let's put this to rest with a simple test. We deliberately delayed content rendering by 4 seconds to force the loading UI to appear first. The verdict? Google's rich-results tool still captured our content perfectly. Even with that 4-second delay, the crawler patiently waited for the complete page to load. So streaming doesn't just improve First Contentful Paint (FCP) for users—it ensures search engines see everything too. Google doesn't rush off like that friend who leaves the party early; it sticks around for the whole show. Your SEO remains intact while your users enjoy faster initial page loads. Win-win.
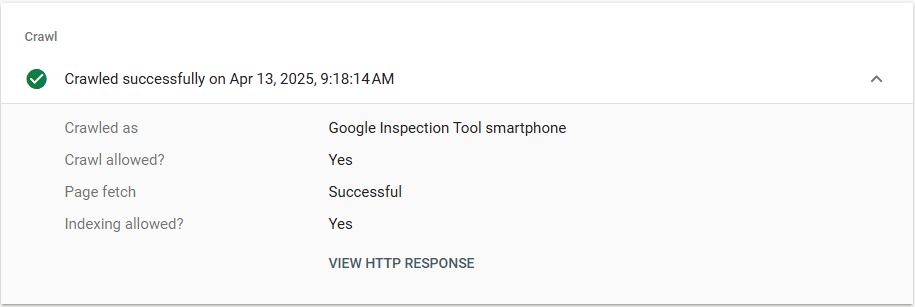
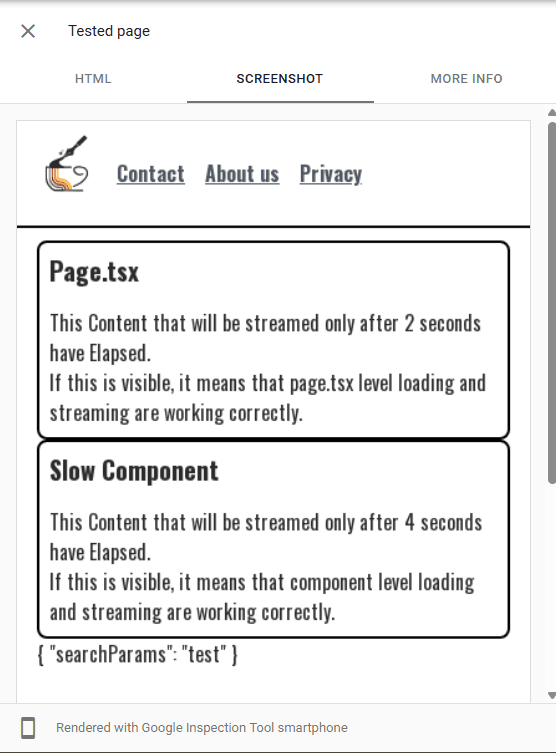
Combining Directory and Component Loading: Watch for Bottlenecks
What happens when you use both directory-level loading (loading.tsx) and component-level Suspense together? Be careful—they don't work in parallel. Here's the catch: component-level streaming only begins after your page component starts rendering. If your page.tsx is stuck loading for 2 seconds, those component-level Suspense boundaries won't even activate until those 2 seconds have passed. Consider this timeline:
- page.tsx takes 2 seconds to load
- SlowComponent needs 2 seconds to fetch its data
- Total time until SlowComponent appears: 4 seconds
Example:
1 "use server"; 2 3 import { Suspense } from "react"; 4 import SlowComponent from "./slowComponent"; 5 import SlowComponentLoader from "./SlowComponentLoader"; 6 7 const delay = (ms: number) => new Promise((res) => setTimeout(res, ms)); 8 9 const Page = async ({ 10 searchParams, 11 }: { 12 searchParams: Promise<{ [key: string]: string | string[] | undefined }>; 13 }) => { 14 await delay(2000); 15 return ( 16 <> 17 <div className="border-2 border-solid border-black rounded-lg p-2 w-fit"> 18 <div className="text-xl font-bold mb-4">Page.tsx</div> 19 <div> 20 This Content that will be streamed only after 2 seconds have Elapsed. 21 </div> 22 <div> 23 If this is visible, it means that page.tsx level loading and streaming 24 is working correctly.{" "} 25 </div> 26 </div> 27 28 <Suspense fallback={<SlowComponentLoader />}> 29 <SlowComponent /> 30 </Suspense> 31 {JSON.stringify(searchParams, null, 2)} 32 </> 33 ); 34 }; 35 36 export default Page;
1 "use server"; 2 const delay = (ms: number) => new Promise((res) => setTimeout(res, ms)); 3 4 const slowComponent = async () => { 5 await delay(2000); 6 return ( 7 <> 8 <div className="border-2 border-solid border-black rounded-lg p-2 w-fit"> 9 <div className="text-xl font-bold mb-4">Slow Component</div> 10 <div> 11 This Content that will be streamed only after 4 seconds(2 of page.tsx + 2 of component) have Elapsed. 12 </div> 13 <div> 14 If this is visible, it means that component level loading and 15 streaming is working correctly. 16 </div> 17 </div> 18 </> 19 ); 20 }; 21 22 export default slowComponent;
Conclusion: Balancing SEO and UX with Next.js Loading Techniques
Strategically leveraging streaming, Suspense, and loading states in Next.js enhances user experience and SEO. Directory-level loading provides efficient route-specific fallbacks, while component-level streaming allows handling slower content without penalizing the faster elements. Be cautious, though; combining these methods can inadvertently prolong load times. Carefully assess and address performance bottlenecks to prevent unintended slowdowns. By balancing these techniques thoughtfully, you achieve quicker initial loads, and improved engagement—ensuring your users remain satisfied and your content is effectively indexed by search engines.